The DBI package in R is an essential tool for working with databases, offering an abstraction layer for connecting and interacting with various database systems, including MySQL, PostgreSQL, SQLite, and others. One of its useful functions, r dbi::sqlappendtable, simplifies the process of appending data to existing tables in a database. In this article, we’ll explore how this function works, its practical uses, and tips for leveraging its power efficiently when working with relational databases in R.
Understanding the Role of r dbi::sqlappendtable
The function r dbi::sqlappendtable belongs to the DBI package in R, which stands for Database Interface. This package defines a set of standards that R follows to interact with relational database management systems (RDBMS). When working with a database, it’s common to update existing tables by appending new rows of data. The r dbi::sqlappendtable function helps generate the correct SQL syntax for appending data to an existing table.
While inserting data into a database is a core task, ensuring that the SQL query is structured correctly, especially with the different formats databases use, can be tedious. The r dbi::sqlappendtable function eliminates the complexity of writing custom SQL queries by automatically generating the SQL commands based on the data provided and the schema of the table.
Syntax and Usage
sqlAppendTable(connection, table, values)
- connection: A DBI connection object that represents an active connection to the database.
- table: The name of the table where data will be appended. It can be a simple table name or a fully qualified name.
- values: A data frame containing the rows of data to be appended to the table.
Let’s break down a simple example where you append data to a database table using sqlAppendTable.
# Load the DBI library
library(DBI)
# Create a connection to an SQLite database
con <- dbConnect(RSQLite::SQLite(), ":memory:")
# Create a simple table
dbExecute(con, "CREATE TABLE employees (id INTEGER, name TEXT, salary REAL)")
# Create a data frame to append
new_employees <- data.frame(
id = c(4, 5),
name = c("Alice", "Bob"),
salary = c(55000, 60000)
)
# Generate the SQL append query
sql_query <- sqlAppendTable(con, "employees", new_employees)
cat(sql_query)
# Append data to the table
dbExecute(con, sql_query)
# Check if the data was added successfully
dbGetQuery(con, "SELECT * FROM employees")
How It Works
In this example:
- A new table employees is created in an in-memory SQLite database.
- A data frame new_employees is defined, containing two new employee records.
- The sqlAppendTable function constructs an SQL query that appends the rows from new_employees to the employees table.
- Finally, the SQL query is executed, and the updated table is retrieved to confirm the data append operation.
What makes sqlAppendTable convenient is its automatic handling of data types and formatting, eliminating the need for manually constructing SQL insert statements.
Advantages of Using r dbi::sqlappendtable
- Automatic SQL Generation: r dbi::sqlappendtable dynamically generates SQL code for appending data, saving time and reducing errors that might occur with manual SQL scripting.
- Compatibility Across Databases: The DBI package provides a consistent interface for different RDBMSs, so whether you are using SQLite, MySQL, or PostgreSQL, the same code works with slight adjustments for database-specific nuances.
- Column Alignment: When appending data, the function ensures that columns in the data frame are properly matched with the table’s schema, preventing mismatches in data types or structure.
- Handling Special Characters: The function also deals with special characters (such as quotes or commas) in the data, making it robust for real-world datasets where such characters often appear.
Practical Use Cases
The r dbi::sqlappendtable function is especially useful in the following scenarios:
- Data Ingestion: When working on data pipelines where new data is regularly fetched from external sources (APIs, files, etc.), you often need to append this data to an existing database table.
- ETL Processes: Extract, Transform, and Load (ETL) operations require regularly appending transformed data to existing tables in the database. sqlAppendTable simplifies the “Load” phase by generating the appropriate SQL.
- Data Archiving: As databases grow, some old data is moved into archival tables. The sqlAppendTable function can be used to transfer data to an archival table efficiently.
- Batch Data Insertion: When large amounts of data need to be inserted into a database table in batches, sqlAppendTable can be used to create and execute SQL queries in chunks, which is more efficient than inserting one row at a time.
Tips for Efficient Use of sqlAppendTable
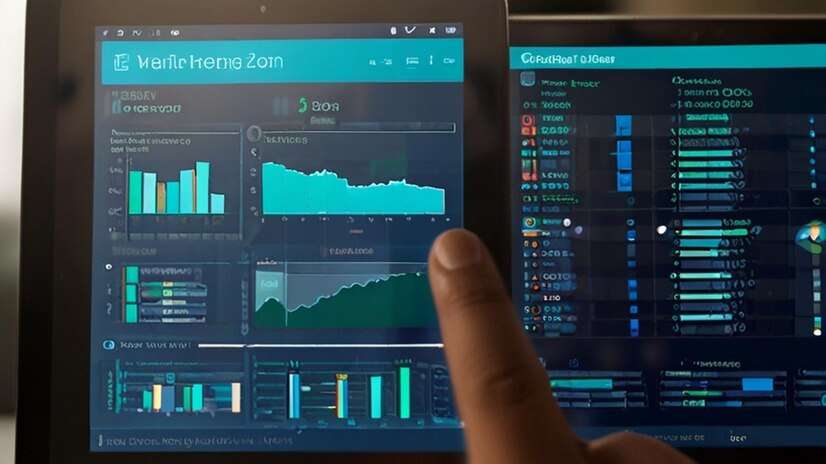
Here are a few tips to make the most of r dbi::sqlappendtable:
- Data Integrity: Before appending data, ensure that the data frame’s column names and types match the structure of the table in the database. If the structure doesn’t match, the generated SQL may fail or result in incorrect data being appended.
- Batch Processing: For very large datasets, it’s advisable to break the data into smaller chunks and append them in batches to avoid overwhelming the database or running into memory issues.
- Error Handling: Wrap your database interactions in error-handling blocks using tryCatch to gracefully handle any exceptions that might arise during the append operation.
- Database Connection Management: Always close database connections after the append operation using dbDisconnect to release resources and avoid connection leaks.
Best Practices When Appending Data
- Data Validation: Always validate the data in your R data frame before appending it to ensure no data type mismatches. This avoids runtime errors during the SQL append operation.
- Primary Key Handling: Ensure that your primary key constraints in the table are respected when appending new rows. If the primary key already exists in the table, the insert will fail.
- Transaction Management: For critical operations, use transactions (dbBegin, dbCommit, dbRollback) to ensure that the append operation is atomic. This way, you can roll back changes if something goes wrong mid-operation.
- Logging: Log SQL operations and results, especially when performing batch appends, to track which data was inserted successfully and which was not.
Conclusion
The r dbi::sqlappendtable function in R provides a streamlined and reliable way to append data to existing tables in a relational database, making it invaluable for anyone working with data pipelines, ETL processes, or any task requiring regular database updates. By abstracting away the complexities of SQL query generation, this function allows you to focus on data analysis and transformation rather than the intricacies of SQL syntax.
With proper validation, batching, and error-handling practices, r dbi::sqlappendtable can significantly improve the efficiency and reliability of your database operations in R. Whether you are an experienced data scientist or a novice working with databases, understanding and using this function effectively can make your workflow smoother and more productive.